Hi Mobile App Developers, Lets take a quick Look at :
Background: We found that Location handling was really one of the areas in android app development where there was some persistent confusion. Trainee staff in our own team had to be explained how to map user requirements to a workable location strategy.
Excluded: Actual code for implementing the fused location which we will look into in a subsequent article.
LEVEL: BEGINNER TO INTERMEDIATE
COVERAGE: Code snippets provided without full working code.
LEVEL: BEGINNER TO INTERMEDIATE
Lets Assume the following use cases for the application of this article:
The client requires that your mobile app show the nearest service provider to him – it could be
a) A Cab Hailing App
b) A Trucking / Commercial Vehicle app
c) A Delivery and Logistic app
d) A Service provider such as a Carpenter / Electrician, Beauty Salon
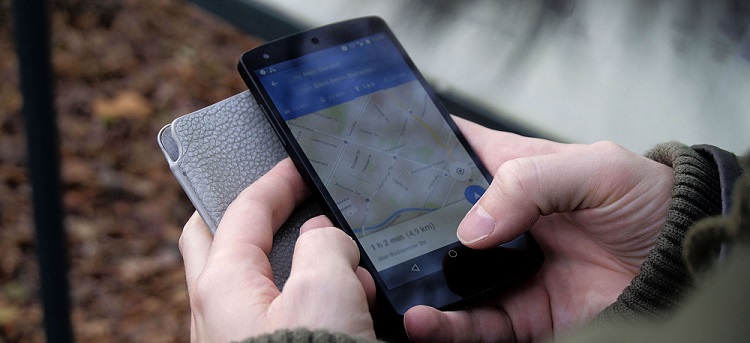
Tackling Location Permissions in Android
If you see carefully, case a), b) , c) are somewhat similar in the sense that a periodic tracking is required whereas case d) is mainly that of retail shops /offices at a single point where periodic tracking of the service provider is not required to know of his location. Just a user initiated update is enough.
How to provide periodic Tracking from the Mobile Phone itself?
So, in the above scenarios a), b), c) a periodic updating of the location – latitude and longitude is required by the app. We’ve generally seen this done by an API which saves the provider’s location periodically into the database and the android activity can retrieve the lat, long of the chosen provider in the activity’s view from the database. The trick is to do this with proper permissions (chosen either on opening app or install etc.) , and with only the required battery consumption.
And this brings us to an important Question, which is:
What is the best way to provide location permissions when developing your Android App?
First up, location was earlier requested through android.location API but since 2014, Fused Location through Google Play Services has been available to get location updates. This is compatible Android 2.3 onwards, so pretty much covers all devices.
Fused location has many advantages and is a recommended way of getting location details according to Google. We assume here that you would know it’s background but if you don’t check out the details here.
So coming back, lets look at how location permission is saved in a typical android phone (Nougat, Marshmallow included).
Situations your Android app must tackle when seeking location updates
A) Either the location is completely off.
OR
B)if the Location setting is on – then
1) Whether GPS option is on (High Accuracy)
2) Whether Networking or Wifi (Low Battery setting) use is On
Fused location provider API (now FusedLocationProviderClient) actually takes care of all these situations and removes the guesswork by automatically changing the appropriate system settings based on desired accuracy and available options /permissions.
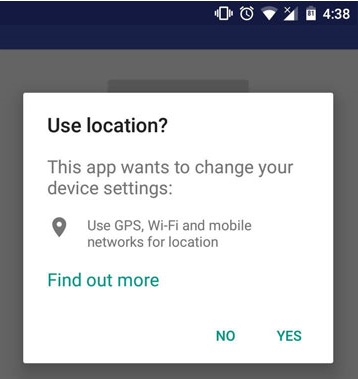
Typical run-time Location Permission Popup in Android
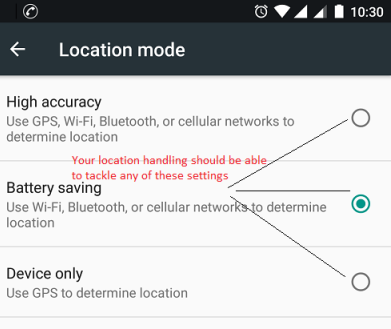
Power Consumption options when choosing Location in Android app
Settings and Permissions Strategy
Keep in mind that many a times networking or low power or what is denoted by COARSE_LOCATION Permission will SUFFICE for your needs. According to Google the accuracy is 50-100 metres for COARSE_LOCATION. If you are in suburb or city in India, i think we can take a worse case scenario of 75-100 metres accuracy.
I would say that this is sufficient for most logistics and delivery app situations such as b) and c) and definitely acceptable for case d). Another aspect that we found was that in many cases, developers had made GPS usage mandatory even when unnecessary.
GPS is mainly necessary for Taxi or Cab hailing apps: where a more frequent update of location as well as direction and speed calculations are required. Practically, one should set Limits of accuracy and / or update as per the business requirements. More frequent or more accurate location settings will lead to greater battery drain.
The App may also provide the user, options to change his / her location settings if he or she wants to – taking full benefit of the location feature. Ideally, it should work with the low power setting also, except where GPS is compulsorily required – such as a cab hailing App.
Therefore, do not restrict the user to use GPS only; unless it’s a business requirement.
As a far as permissions are concerned if location is required regularly, it would be better to take permission on install but switch location services off whenever not needed. If it is infrequent or based on a specific activity or event then you can give permissions at Runtime. In order to use the location services provided by Google Play Services Fused Location Provider, connect your app using the Settings Client, check the current location settings and prompt the user to enable the required settings if needed.
Steps in getting Location Updates in Fused Location (Location services Android Tutorial)
1. Setting the Permission
Import Google Play services from Android SDK (../sdk/extras/google/google_play_service/libproject/google-play-services_lib) as a library in your application
{
manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.google.android.gms.location.sample.locationupdates"
/manifest
}
2. Create a Location Services Client
private FusedLocationProviderClient mFusedLocationClient;
// ..
@Override
protected void onCreate(Bundle savedInstanceState) {
// ...
mFusedLocationClient = LocationServices.getFusedLocationProviderClient(this);
}
3. Getting the Last known Location
mFusedLocationClient.getLastLocation()
.addOnSuccessListener(this, new OnSuccessListener
@Override
public void onSuccess(Location location) {
// Got last known location. In some rare situations this can be null.
if (location != null) {
// Logic to handle location object
}
}
});
Note that null handling has to be done as in some scenario location may not be returned. Also, the above step is not really necessary if we’re requiring periodic location updates.
To get periodic location updates, and in our own control we need to set up a Location request client and get location requests from it at pre-configured periodic intervals.
And to do this you have to define all these configuration settings. Which brings us to Step 4.
4. Set Update interval, Fastest update interval and Priority Configuration for Location Updates.
In this case lets assume we have a case where infrequent location updates are fine – say every 10 minutes is more than enough. Also lets assume a very high accuracy is not required and we can do with 100 metre error. Typical settings in such as scenario would probably be like this:
protected void createLocationRequest() {
LocationRequest mLocationRequest = new LocationRequest();
mLocationRequest.setInterval(900000);
mLocationRequest.setFastestInterval(600000);
mLocationRequest.setPriority(LocationRequest.PRIORITY_BALANCED_POWER_ACCURACY);
}
So as you can see – we’re defining these settings in the LocationRequest object just created. We have the setFastestInterval as upper limit for any location update (which other apps may also be getting ) to be used by you. So for us since 10 mins is the minimum and we can do with 15 minutes as well – we’ve kept the values accordingly (measured in milliseconds).
After creating the locationRequest – get the current location to begin with:
LocationSettingsRequest.Builder builder = new LocationSettingsRequest.Builder()
.addLocationRequest(mLocationRequest);
Apart from this, you can also get the Current Location Settings, prompt user to change settings if required (an optional step in most cases) as explained here. NOW you are ready to get the regular Location updates which is required for Tracking.
5. Getting Regular Location updates from Fused Location.
The API updates your app periodically with the best available location, based on the currently-available location providers such as WiFi and GPS (Global Positioning System). The accuracy of the location is determined by the providers, the location permissions you’ve requested, and the options you set in the location request.
@Override
protected void onResume() {
super.onResume();
if (mRequestingLocationUpdates) {
startLocationUpdates();
}
}
private void startLocationUpdates() {
mFusedLocationClient.requestLocationUpdates(mLocationRequest,
mLocationCallback,
null /* Looper */);
}
So startLocationUpdates is the main method which is passing the LocationRequest object and Callback.
mRequestingLocationUpdates is a boolean flag which is used to indicate if location requests are ON or OFF by the user of the App.
Now the LocationCallback interface also needs to be implemented which actually gets the Latitude and Longtitude.
private LocationCallback mLocationCallback;
// ...
@Override
protected void onCreate(Bundle savedInstanceState) {
// ...
mLocationCallback = new LocationCallback() {
@Override
public void onLocationResult(LocationResult locationResult) {
for (Location location : locationResult.getLocations()) {
// Update UI with location data
// ...
}
};
};
}
Stopping or Pausing a Location Request
This is an important option which can help reduce power consumption to stop getting location updates when not needed. For example, if it’s a regular saving of location in database but when your vehicle is stopping or in rest – on click of your Rest / Shift Completed button, the location updates could stop as there is no need to track you. This could even be time based.
@Override
protected void onPause() {
super.onPause();
stopLocationUpdates();
}
private void stopLocationUpdates() {
mFusedLocationClient.removeLocationUpdates(mLocationCallback);
}
Note: The important thing to be kept in mind is that the above examples show location requests based on a specific activity but if your requirement is such that tracking continues even if application is in background then you need to use a background service and get the current location. In that scenario , you do NOT need to request Location updates as the background service itself can be run periodically and just get the current location. Further , here the settings need not be configured to Reflect the Fastest interval and just the Accuracy to BALANCED POWER would be enough.
Another option could be to use PendingIntent as explained here to get location in background cases.
If you’re looking to build a Location, GPS tracking, Logistics or Cab hailing app , maybe our mobile app development company based out of Delhi, can help you. Just reach out to us and we’ll be happy to provide a free consultation.